
Java Inventory Application
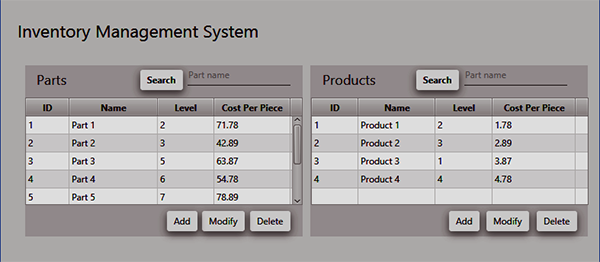
About the Project
In this report I present a comprehensive analysis of a Java desktop application designed to modernize the inventory management system for a small vehicle manufacturing company as part of a school project. Developed using IntelliJ IDE and JavaFX, the application is architected around the Model-View-Controller (MVC) design pattern and includes object-oriented design principles such as inheritance, encapsulation, abstraction, and more.
Challenge
The objective of this project was to develop a Java desktop application that incorporates key object-oriented design principles
Scenario and Requirements
This school project addresses the needs of a small manufacturing organization that has outgrown its rudimentary, spreadsheet-based inventory management system. The organization had been manually entering inventory data, including additions and deletions, into a spreadsheet program. The limitations of this approach became evident as the organization grew, necessitating a more sophisticated, automated solution for inventory management. This section is a very brief summary of the requirements and core competencies of this project.
Planning The Application
Classes and Interfaces
The graduate designs software solutions with appropriate classes, objects, methods, and interfaces to achieve specific goals.
Object-Oriented Principles
The graduate implements object-oriented design principles (e.g., inheritance, encapsulation, and abstraction) in developing applications for ensuring the application’s scalability.
Application Development
The graduate produces applications using Java programming language constructs to meet business requirements.
Exception Handling
The graduate incorporates simple exception handling in application development for improving user experience and application stability.
User Interface Development
The graduate develops user interfaces to meet project requirements.
UML Diagram and Class Structure
In this section I will discuss the architectural blueprint of the Java desktop application as represented by the Unified Modeling Language (UML) diagram and I will summarize the Object Oriented Programming concepts implemented in the project. The diagram outlines the roles and relationships between classes, methods, and attributes, offering a clear view of the system’s structure. It’s particularly useful for understanding how the classes fit into the Model-View-Controller (MVC) architecture. In MVC, classes in the ‘Model’ handle the core business logic, while those in the ‘View’ manage the user interface. The ‘Controller’ classes act as intermediaries, linking the Model and View. The UML diagram helps to visualize these roles and interactions, making it easier to grasp the flow of data and control within the application.
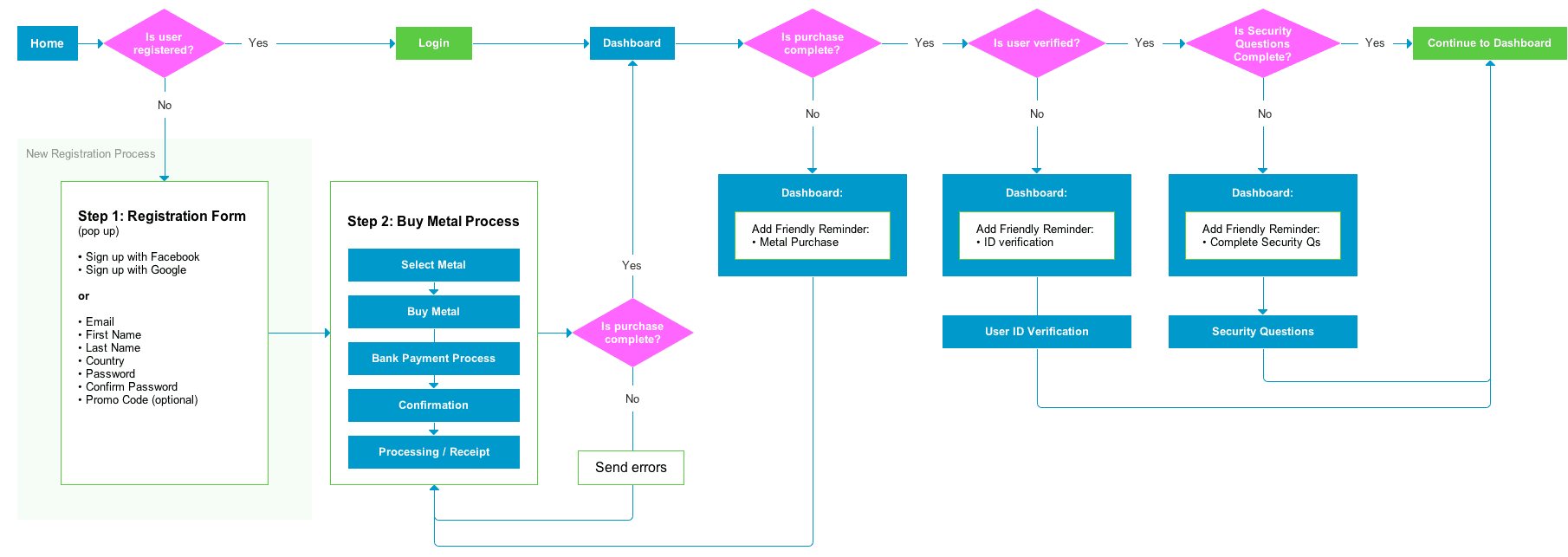
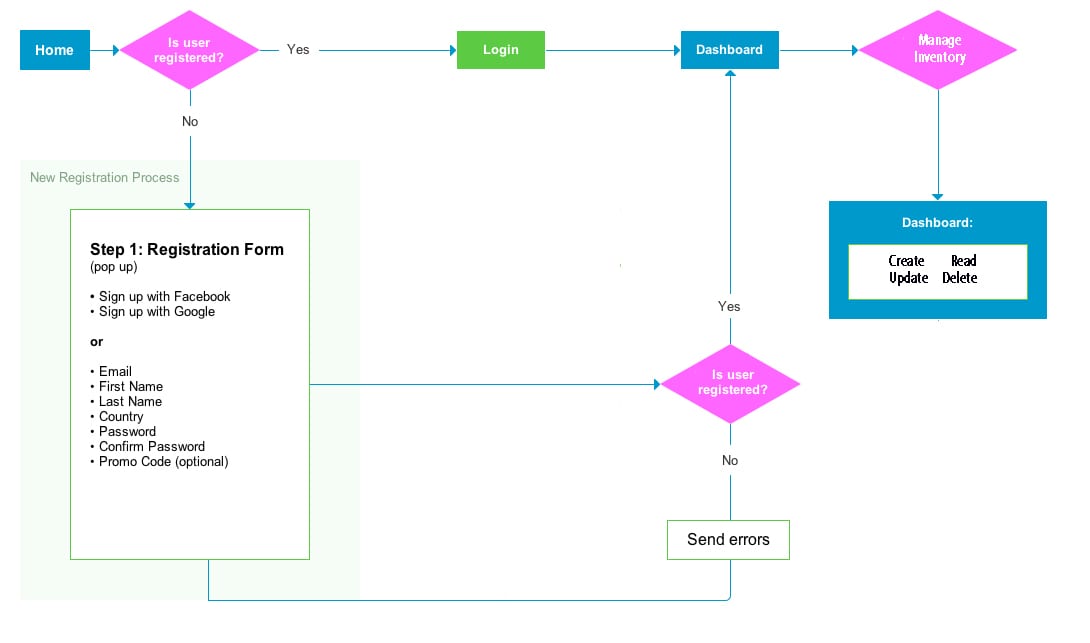
Inventory Class
The Inventory
class manages the overall inventory of parts and products. It provides methods for adding, deleting, and modifying parts and products. It acts as a mediator between the Main
class and the Part
and Product
classes, ensuring data integrity and facilitating various functionalities.
Attributes
allParts
: An observable list that holds all thePart
objects in the inventory.allProducts
: An observable list that holds all theProduct
objects in the inventory.-
Methods
addPart(Part newPart): This method allows for the addition of a new part to the inventory. It takes a
Part
object as an argument and adds it to theallParts
list.
addProduct(Product newProduct): Similar to addPart
, this method allows for the addition of a new product to the inventory. It takes a Product
object as an argument and adds it to the allProducts
list.
deletePart(Part selectedPart): This method removes a selected part from the inventory. It takes the Part
object to be deleted as an argument and removes it from the allParts
list.
deleteProduct(Product selectedProduct): This method removes a selected product from the inventory. It takes the Product
object to be deleted as an argument and removes it from the allProducts
list.
lookupPart(int partID) and lookupPart(String partName): These are overloaded methods that allow for the searching of parts either by their ID or name. They return a list of parts that match the search criteria.
lookupProduct(int productID) and lookupProduct(String productName): Similar to lookupPart
, these overloaded methods allow for the searching of products either by their ID or name. They return a list of products that match the search criteria.
Part Class (Abstract)
The Part
class serves as the base class for all types of parts in the inventory system. It is designed as an abstract class, embodying the object-oriented principle of abstraction. This class defines the common attributes and methods that are shared by all types of parts, whether they are manufactured in-house or outsourced.
Attributes
id
: Unique identifier for each part.name
: Name of the part.price
: Price of the part.stock
: Inventory level or stock of the part.min
: Minimum allowable inventory level.max
: Maximum allowable inventory level.
Methods
getID()
,getName()
,getPrice()
, etc.: Getter methods for retrieving the attributes.setID(int id)
,setName(String name)
,setPrice(double price)
, etc.: Setter methods for modifying the attributes.
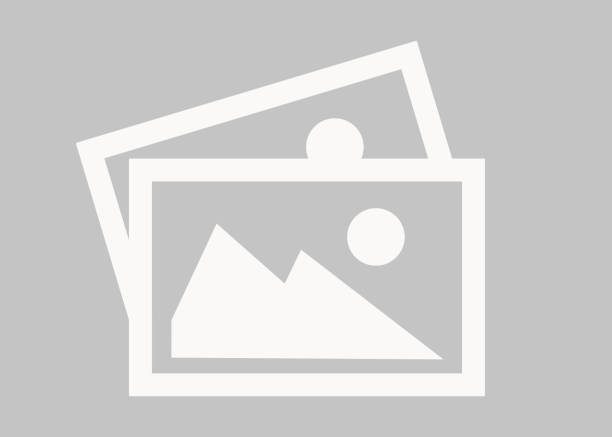
InHouse Part – Class Extension
The InHouse
class is a concrete class that extends the Part
class. It adds an additional attribute, machineID
, specific to in-house parts. This class inherits common functionalities from the Part
class while adding its own unique features.
Additional Attributes
machineID
: Identifier for the machine that manufactured the part.
Additional Methods
getMachineID()
: Getter method for retrieving the machine ID.setMachineID(int machineID)
: Setter method for modifying the machine ID.
Outsourced Part – Class Extension
The Outsourced
class also extends the Part
class and adds an additional attribute, companyName
, to specify the name of the company from which the part is sourced. Like the InHouse
class, it inherits common functionalities from the Part
class.
Additional Attributes
companyName
: Name of the company from which the part is outsourced.
Additional Methods
getCompanyName()
: Getter method for retrieving the company name.setCompanyName(String companyName)
: Setter method for modifying the company name.
Product Class
The Product
class represents the products in the inventory, each of which can contain zero or more associated parts. It has attributes like productID
, name
, price
, stock
, min
, and max
, similar to the Part
class. It also maintains a list of associated parts, allowing for the addition and removal of parts.
Attributes
id
: Unique identifier for each product.name
: Name of the product.price
: Price of the product.stock
: Inventory level or stock of the product.min
: Minimum allowable inventory level.max
: Maximum allowable inventory level.associatedParts
: A list containing the parts that are associated with the product.
Methods
getID()
,getName()
,getPrice()
, etc.: Getter methods for retrieving the attributes.setID(int id)
,setName(String name)
,setPrice(double price)
, etc.: Setter methods for modifying the attributes.addAssociatedPart(Part part)
: Method to add a part to the list of associated parts.deleteAssociatedPart(Part part)
: Method to remove a part from the list of associated parts.getAllAssociatedParts()
: Method to retrieve the list of all associated parts.
Roboto
A B C D E F G H
abcdefghijklm
nopqrstuvwxyz
1234567809
Roboto has a dual nature. It has a mechanical skeleton and the forms are largely geometric. At the same time, the font features friendly and open curves. While some grotesks distort their letterforms to force a rigid rhythm, Roboto doesn't compromise, allowing letters to be settle in to their natural width. This makes for a more natural reading rhythm more commonly found in humanist and serif types.
Light + Regular
Bb
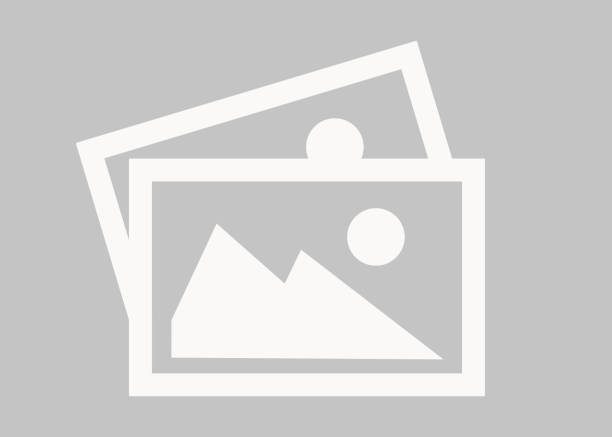
Object Oriented Programming Concepts Implemented
- Inheritance: Inheritance allows a class to use methods and fields of another class. In this project, the
InHouse
andOutsourced
classes inherit from thePart
class. This setup promotes code reuse and establishes a clear hierarchical structure, where common attributes like ID, name, and price are inherited from the base class.
- Encapsulation: Encapsulation restricts direct access to some of an object’s components, which can prevent the accidental modification of data. In classes like
Product
andPart
, all attributes are private, and public getter and setter methods are provided. This ensures data integrity by controlling how data can be accessed or modified.
- Polymorphism: Polymorphism allows objects to be treated as instances of their parent class, enabling a single interface to control different types. The system uses this principle to handle both
InHouse
andOutsourced
parts through methods that operate on thePart
type, offering a unified way to manage different types of parts.
- Abstraction: Abstraction involves hiding the complex reality while exposing only the essential parts. The
Initializable
interface implemented by controllers likeAddProduct
andModifyProduct
provides a common methodinitialize
that each class overrides for UI setup, ensuring a consistent initialization pattern across controllers.
- Composition: Composition allows a class to contain instances of one or more classes as members. The
Product
class demonstrates this by containing a list ofPart
objects, effectively allowing aProduct
to be composed of multiple parts and managing their lifecycle.
- Association: Association is a relationship where all objects have their own lifecycle and there is no owner. The
Product
andPart
classes have a many-to-many association managed through methods likeaddAssociatedPart
anddeleteAssociatedPart
, allowing for complex relationships between products and parts.
- Method Overloading: Method Overloading allows multiple methods to have the same name but different parameters. The
lookupPart
method is overloaded to accept either a string for the name or an integer for the ID, offering multiple ways to perform the same action.
- Singleton Pattern: The Singleton Pattern restricts a class from instantiating multiple objects. It is used in the
Inventory
class to ensure a single point of access to inventory data, which is essential for maintaining data consistency across the application.
Controller Logic
In the Model-View-Controller (MVC) architecture, the Controller acts as a sophisticated mediator that orchestrates the interaction between the Model and the View. Within the scope of this inventory management system, the Controller assumes a multi-faceted role that goes beyond basic event handling. It is responsible for a range of complex actions, including the addition, modification, and deletion of parts and products in the Inventory.
Technically, the Controller is implemented as a set of Java classes equipped with methods that are annotated with JavaFX’s FXML to bind them to specific UI events. These methods listen for triggers originating from the View—such as button clicks, text input, or table selections—and execute corresponding logic. This can involve validating user input, invoking methods from the Model to manipulate the underlying data, and updating the View to reflect these changes.
For instance, methods like onActionAddPart
or onActionSaveProduct
not only capture the event but also perform data validation, update the Model by calling its API, and then refresh the View. The Controller also handles conditional logic, such as determining whether a part is ‘InHouse’ or ‘Outsourced’, and dynamically adjusts the available UI components accordingly.
Moreover, the Controller employs advanced JavaFX features like ObservableLists and Property Binding to keep the View in sync with the Model. This ensures that any changes to the data are immediately reflected in the UI, providing a responsive and intuitive user experience.

Main Menu Controller
Overview
The MainMenu
class serves as the primary controller for the main menu of the inventory management system. It handles various functionalities, including displaying parts and products, navigating to other pages, and performing CRUD operations (Create, Read, Update, Delete) on parts and products. The class implements the Initializable
interface, which allows it to run initialization logic after the UI components are loaded.
Attributes
- FXML Components: The class uses several FXML-annotated attributes to bind to the UI components, such as tables (TableView), columns (TableColumn), and text fields (TextField).
- Stage Object: A
Stage
object is used to manage scene changes.
Methods
- changeScenes(String fxmlDocument, ActionEvent event): A utility method for changing scenes. It takes the name of the FXML document and the event source to switch to a new scene.
- onActionAddPart(ActionEvent event): Opens the ‘Add Part’ page.
- onActionModifyPart(ActionEvent event): Opens the ‘Modify Part’ page for the selected part. It uses the sendPart() method from the ModifyPart controller to send the selected part for modification.
- onActionDeletePart(ActionEvent actionEvent): Deletes the selected part after user confirmation.
- onPartSearchClick(MouseEvent mouseEvent): Clears the search text field when clicked.
- onPartKeyRelease(KeyEvent keyEvent): Searches for parts as the user types in the search field.
- onPartSearch(ActionEvent actionEvent): Executes a search when the user presses Enter.
- onActionAddProduct(ActionEvent event): Opens the ‘Add Product’ page.
- onActionModifyProduct(ActionEvent event): Opens the ‘Modify Product’ page for the selected product. It uses the sendProduct() method from the ModifyProduct controller to send the selected product for modification.
- onActionDeleteProduct(ActionEvent actionEvent): Deletes the selected product after user confirmation.
- onProductSearchClick(MouseEvent mouseEvent): Clears the search text field when clicked.
- onProductKeyRelease(KeyEvent keyEvent): Searches for products as the user types in the search field.
- onProductSearch(ActionEvent actionEvent): Executes a search when the user presses Enter.
- onActionExit(ActionEvent actionEvent): Exits the application.
Initialization
- initialize(URL url, ResourceBundle resourceBundle): Initializes the tables for parts and products. It sets the data and sorts it by ID.
Error Handling
The class includes various runtime error handling mechanisms, often using Alert
dialogs to inform the user about issues such as not selecting a part or product before modification or deletion.
Initial Application Functionality
Once we created the initial crud application using MAMP, we began to create the functions of the pages and flush out the initial application.
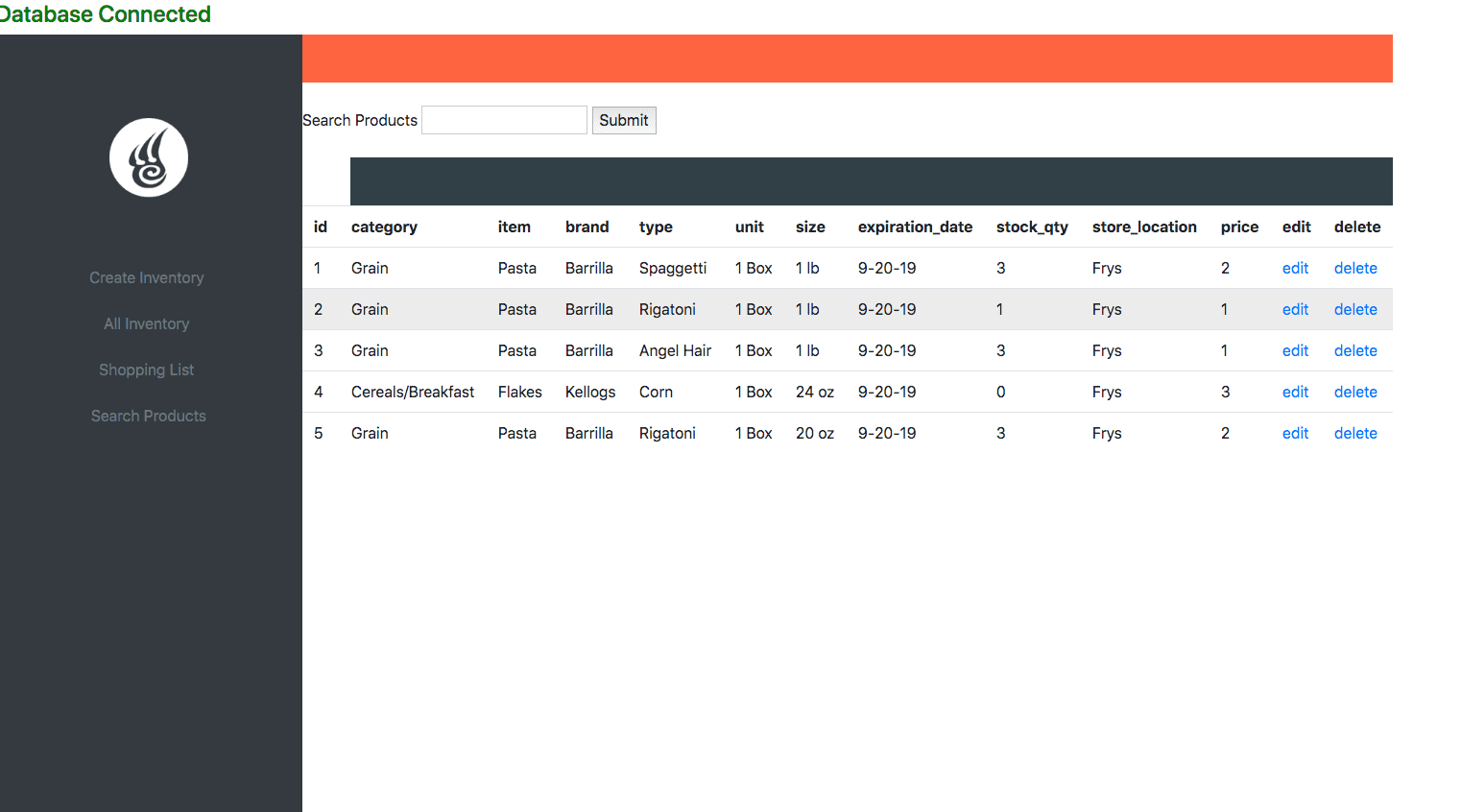
Website Design
Mobile Responsive
To begin the website design process, I did some research and created a mood board for the application. The issue of creating a dashboard was easy to envision for desktop, but the challenge of form tables and user experience grew more complex quickly. we did research on how to make bootstrap 4 table responsive and realized our grid wold need adjusting .